How to: get the node index of an element
Javascript has no in-built way of returning the node index of an element from amongst its siblings.
That is, if you have a of 5 s, and you click the second of them, how can you get JS to know it was the second?
The trick is to count the node's previous siblings. If you count them then add one, you'll have the node index of your element. Here's a jQuery plugin for this:
1jQuery.fn.nodeIndex = function() {
2 return $(this).prevAll().length + 1;
3};
Here's how you'd use it:
1$('ul#myUL li').click(function() {
2 alert("You clicked
3});
This is a good opportunity to show just how much time and code frameworks like jQuery (motto: "write less, do more") can save. Here's the same thing in native Javascript:
1function nodeIndex(node) {
2 if (node && typeof node == 'object') {
3 var siblings = node.parentNode.childNodes;
4 var counter = 0;
5 for(var i=0; i 6 if (siblings[i].tagName != undefined) 7 if (siblings[i] === node) break; 8 else 9 counter++; 10 } 11 return counter; 12 } 13}
And to use this:
1var lis = document.getElementById('myUL').getElementsByTagName('li');
2for (var k=0; k 3 lis[k].onclick = function() { 4 alert(nodeIndex(this)); 5 } 6}
The whole point of frameworks like jQuery is to automate industrial, utility tasks such as this.
post a commentMatch elements via REGEXP in jQuery
One of the odd omissions from jQuery's famous selectors is the ability to filter by regular expression. The usual work-around is to iteratively interrogate each chain element's text in a callback function inside .filter(), but this is a little clunky and feels unnecessasry.
Mitya to the rescue. I've just posted a plugin and core extension which allows precisely this, both via a pseudo selector, :regexp, and via a method, .regexp().
Head over here to download, get usage info or view a demo.
So for example, to use the pseudo selector:
$('selector:regexp(/pattern/)') //etc
or, to achieve the same effect with the method:
$(selector).regexp(/pattern/) //etc
Note that, to use the pseudo method, you'll need to double-escape any characters that need escaping. This is because you're specifying a REGEXP pattern inside a string.
post a commentGD barchart generator
Just posted this in the script section. It's a script for generating bespoke barcharts. It's powered by PHP's superb GD library, which I've long loved using and which allows the creation of dynamic images via PHP.
Head over here to download, get usage info or view a demo.
The script gives you full control over how your chart looks, including the data displayed, the axis increments, the colours, font angle and much more.
post a commentTable sort - now REGEXP friendly
Just modified my animated table sort plugin to allow sorting on a regular expression match against the content of each
This would be hugely useful if, say, you had a table showing product categories and, for each, the number of products therein, in brackets, e.g. "Lawnmowers (15)".
You can also specify an index of the returned matches array to use for the sort, in case your REGEXP pattern returns more than one match or specifies a sub-pattern.
Head over here to download, get usage info or view a demo.
post a commentHello, world
I'm a London-based Javascript specialist, focussing on native JS, jQuery, XSL and related technologies.
Latest content
My projects/writings
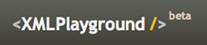
XMLPlayground.com - a sandbox for XML development, including (E)XSLT, XQuery, DTD, Schema and more
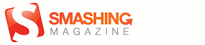
- Ten Oddities and Secrets of JavaScript
- Commonly Confused Bits of jQuery
- Image Manipulation with jQuery and PHP-GD
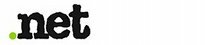
- Get Serious with JavaScript: Learn RegExp (in print copies only)
- Catching Up with jQuery's Newer Versions (submitted for print...)
Archive
-
2012
-
Aug
- > Article by me in next issue of .NET
-
Jul
- > The magic of JavaScript's apply()
- > REGEX round-up: two issues...
-
Jun
- > What have you tried?
- > XMLPlayground.com - my new project
-
May
- > A look at native JSON support in ECMA5
-
Apr
- > Distinguishing real events from simulated events
- > Non-AJAX use for jQuery's deferred objects
-
Mar
- > JSON-P: what it is, what it's not and how it works
- > JavaScript getters and setters: varying approaches
-
Feb
- > ECMAScript 5: a revolution in object properties
- > A friendly alternative to currying
- > Presenting XMLTree v.2.0
-
Jan
- > Numberfy: add line numbers to your textareas
- > JSON and PHP: formatting and validating
-
-
2011
-
Dec
- > Being Firebug, pt2: which rules, which elements?
- > Being Firebug, pt1: reading CSS in Javascript
-
Nov
- > Additions to XML tree; jQuery XML parsing
- > BAPS (browser and plugin sniffer)
- > Roundup: animated table sort / browser sniffing
-
Oct
- > Annoyances with client-side XSL transformation
- > XML Tree - visualise and traverse your XML
-
Sep
- > Fiddler - when debugging needs to go nuclear
- > My article in .NET / update to Dropdown
-
Aug
- >
- > Using Dropdown as a fancy form field
- > The site gets a spring-clean
-
Jul
- > REGEX article by me in .NET magazine
-
Jun
- > Understanding multi-line mode for JS REGEX
- > On-the-fly conversion of Flash to image for iOS
-
May
- > My new article on JS oddities and well-kept secrets
- > Understanding Javascript self executing functions
-
Apr
- > Twisted logic: understanding truthy and falsy
- > New Smashing article by me on image manip
-
Mar
- > Update to lightbox and lightbox dialog
- > Update to date calculator script
- > Bizarre webkit bug with 'typeof'
-
Feb
- > Simulating REGEX look-behinds in JavaScript
- > Smashing jQuery out now (I was technical editor...)
- > Bouncy text plugin
-
Jan
- > Uber-customisable video carousel
-
-
2010
-
Dec
- >
-
Nov
- > XML-to-JSON convertor and remapper
- > Table sorter: toggling sort direction
-
Oct
- > Blockster - fixed for jQuery v1.4.3
- > Like my work? Remember it's free...
- > JAWS: news ticker and mini accordion
- > Sliding block puzzle game in Javascript
-
Sep
- > Round-up: IE6 radio bug; updated Dropdown...
- > Talking to the zebra: new comments system
- > Blockster update: controlling it with navigation
- > My new carousel - now documented!
-
Aug
- > Mitya's lovely new carousel
- > How to: detect internet connection in Javascript
- > jQuery article by Mitya on SmashingMagazine.com
-
Jul
- > Spraycan - vandalise your page
- > Table sort update - sorting on child element
- > jQuery drop-down selector
- > Blockster transition effect
-
Jun
- > How to: get the node index of an element
- > Match elements via REGEXP in jQuery
- > GD barchart generator
- > Table sort - now REGEXP friendly
- > UI for Postcode Anywhere address look-up
- > Animated table sort
- > Ajaxify - re-route your forms via AJAX
- > curveMe - cross-browser curvy corners
- > Calculate time between two dates
-
May
- > Calendar and date-picker
- > Domscripter - reduce your DOM-scripting code
- > MSThis - simulate W3C events in IE
- > Lightbox and lightbox dialog
- > Hello, world
-
Other stuff, er...
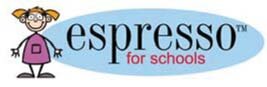
...I lead front-end development at London-based Espresso Education. More info...

...I'm part of the O'Reilly Blogger Review Scheme